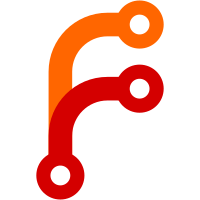
- refactor: change default import alias - feat: add icons to breadcrumbs - fix: add `site_name` og tag - feat: hover on feedback for `Link`s and `TableofContentsHeadings` - fix: add `external` to `SocialIcons`
87 lines
2.8 KiB
Text
87 lines
2.8 KiB
Text
---
|
|
import '../styles/global.css'
|
|
import '../styles/katex.css'
|
|
|
|
import { SITE } from '@/consts'
|
|
import { ViewTransitions } from 'astro:transitions'
|
|
|
|
interface Props {
|
|
title: string
|
|
description: string
|
|
image?: string
|
|
}
|
|
|
|
const canonicalURL = new URL(Astro.url.pathname, Astro.site)
|
|
|
|
const { title, description, image = '/static/twitter-card.png' } = Astro.props
|
|
---
|
|
|
|
<meta charset="utf-8" />
|
|
<meta name="viewport" content="width=device-width,initial-scale=1" />
|
|
<meta name="generator" content={Astro.generator} />
|
|
|
|
<link rel="canonical" href={canonicalURL} />
|
|
<link rel="sitemap" href="/sitemap-index.xml" />
|
|
|
|
<title>{title}</title>
|
|
<meta name="title" content={title} />
|
|
<meta name="description" content={description} />
|
|
|
|
<link rel="apple-touch-icon" sizes="180x180" href="/apple-touch-icon.png" />
|
|
<link rel="icon" type="image/png" sizes="32x32" href="/favicon-32x32.png" />
|
|
<link rel="icon" type="image/png" sizes="16x16" href="/favicon-16x16.png" />
|
|
<link rel="manifest" href="/site.webmanifest" />
|
|
<link rel="mask-icon" href="/safari-pinned-tab.svg" color="#121212" />
|
|
<meta name="msapplication-TileColor" content="#121212" />
|
|
<meta name="theme-color" content="#121212" />
|
|
|
|
<meta property="og:type" content="website" />
|
|
<meta property="og:url" content={Astro.url} />
|
|
<meta property="og:site_name" content={SITE.TITLE} />
|
|
<meta property="og:title" content={title} />
|
|
<meta property="og:description" content={description} />
|
|
<meta property="og:image" content={new URL(image, Astro.url)} />
|
|
|
|
<meta property="twitter:card" content="summary_large_image" />
|
|
<meta property="twitter:url" content={Astro.url} />
|
|
<meta property="twitter:title" content={title} />
|
|
<meta property="twitter:description" content={description} />
|
|
<meta property="twitter:image" content={new URL(image, Astro.url)} />
|
|
|
|
<ViewTransitions />
|
|
|
|
<script is:inline>
|
|
function setDarkMode(document) {
|
|
const getThemePreference = () => {
|
|
if (
|
|
typeof localStorage !== 'undefined' &&
|
|
localStorage.getItem('theme')
|
|
) {
|
|
return localStorage.getItem('theme')
|
|
}
|
|
return window.matchMedia('(prefers-color-scheme: dark)').matches
|
|
? 'dark'
|
|
: 'theme-light'
|
|
}
|
|
const isDark = getThemePreference() === 'dark'
|
|
document.documentElement.classList[isDark ? 'add' : 'remove']('dark')
|
|
|
|
if (typeof localStorage !== 'undefined') {
|
|
const observer = new MutationObserver(() => {
|
|
const isDark = document.documentElement.classList.contains('dark')
|
|
localStorage.setItem('theme', isDark ? 'dark' : 'theme-light')
|
|
})
|
|
observer.observe(document.documentElement, {
|
|
attributes: true,
|
|
attributeFilter: ['class'],
|
|
})
|
|
}
|
|
}
|
|
|
|
setDarkMode(document)
|
|
|
|
document.addEventListener('astro:before-swap', (ev) => {
|
|
// Pass the incoming document to set the theme on it
|
|
setDarkMode(ev.newDocument)
|
|
})
|
|
</script>
|